In this article, you’ll learn how to remove leading and trailing whitespace in XPath using the normalize-space() function for cleaner and more accurate text matching.
What is normalize-space in Selenium?
Normalize-space is a very useful function in XPath for whitespace normalization when you build it concerning some string or keyword to use it in the Selenium test script, and it has leading or trailing intermediate repeating white space. The normalize-space() function will strip such leading and trailing white space. In Selenium webdriver, very often we use keyword references in building XPath. If there is no good reference to build xapth, then it is mandatory to use such keywords as reference.
Syntax of normalize-space() in XPath
normalize-space([string])
- [string] – Optional. If provided, it returns the string with leading, trailing, and multiple internal spaces reduced to a single space.
- If no argument is passed, it applies to the current context node’s string value.
You can look at my post describing different ways to build XPath.
Example of whitespace normalization in XPath
Here I am presenting one example where we will use the normalize-space function to build xpath and use it in the Selenium webdriver test script.
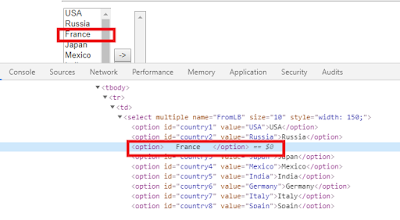
Look at the above image. You can see that the label France contains leading and trailing space. Now, if you want to use the keyword France in XPath building, you must trim the leading and trailing white-space from a string. Otherwise, it won’t work for you. So here, the Normalize-space() method will help you to strip leading and trailing whitespace.
Example: Using normalize-space() in XPath
Let’s say you have the following HTML:
<div> Welcome to Selenium </div>
If you try to match the exact text with extra spaces, it might fail. Instead, use normalize-space():
//div[normalize-space(.) = 'Welcome to Selenium']
It will match the text content of an HTML element with a given value while ignoring all redundant or extra spaces in the target string.
What is the difference between text and normalize-space in XPath?
The text() method will match the text of an element. However, the normalize-space() function will remove leading and trailing white space from a string, and then it will match the text.
Selenium test script for normalizing space
Complete selenium script demonstrates the usage of normalize-space as below.
package test;
import java.util.concurrent.TimeUnit;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.Select;
public class basic {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver","D:\chromedriver.exe");
WebDriver driver = new ChromeDriver();
driver.manage().window().maximize();
driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS);
driver.get("https://only-testing-blog.blogspot.com/2014/01/textbox.html");
driver.findElement(By.xpath("//*[@id="check3"]")).click();
//Used Normalize-space to strip leading and trailing space.
driver.findElement(By.xpath("//*[normalize-space(text())='France']")).click(); //working copy
}
}
In the above given normalized space in the selenium example, you can see that the normalize-space function is used in XPath building to strip leading and trailing white space.
Hi, I’m Aravind, a seasoned Automation Test Engineer with 17+ years of industry experience. I specialize in tools like Selenium, JMeter, Appium, and Excel automation. Through this blog, I share practical tutorials, tips, and real-world insights to help testers and developers sharpen their automation skills.