Learn how to perform a right click in Playwright, with step-by-step instructions and code examples.
In playwright automation, you frequently need to simulate a mouse right-click action on an element to test context menus or special actions. You can use the click() method with a modifier for the mouse button to perform a right-click action.
What is a Right Click in Web Automation?
A right-click, also known as a context click, usually opens a custom menu or triggers a special action on a web page. It is essential to automate this action when you want to test a custom context menu, simulate user behavior, or validate a functionality that appears only on right-click.
How to Right-Click in Playwright
Playwright has a built-in click() method to simulate left-click action. But you can easily change it to a right-click.
Here is a syntax to perform a right-click action in Playwright using the click() method.
Right-click action syntax
await page.click(selector, { button: 'right' });
- selector: The CSS selector of the element you want to right-click on
- button: ‘right’: Tells Playwright to perform a right-click instead of the default left-click
Playwright Right Click Example
Let’s walk through a complete example of how to use right-click in Playwright using JavaScript.
const { test, expect } = require('@playwright/test');
test('Example of right-click action in Playwright', async ({ page }) => {
// Navigate to your test page
await page.goto('https://swisnl.github.io/jQuery-contextMenu/demo.html');
// Right click on the element
await page.click('.context-menu-one', { button: 'right' });
//Wait for 5 seconds to visually see context menu.
await page.waitForTimeout(5000);
//Click on context menu item.
await page.getByRole('listitem').filter({ hasText: 'Cut' }).click();
});
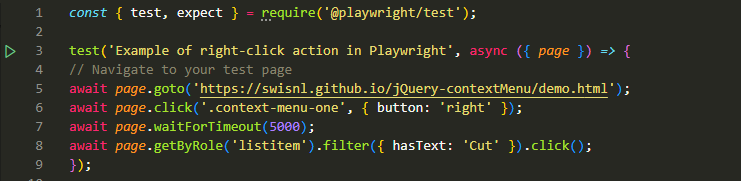
Things to Keep in Mind while performing a right-click
- Some elements may block right-click through JavaScript. In such cases, you can use force: true if needed.
- Test across multiple browsers (Chromium, Firefox, WebKit) for consistency.
- Make sure context menus load correctly and don’t rely on browser-native menus unless necessary.
Use Case: When Should You Use Right Click in Testing?
You should use right-click in Playwright when your web application has:
- Custom context menus
- File/folder explorer interfaces
- Interactive dashboards with right-click options
- Rich text editors or design tools
Final Words
Whether you’re testing a custom context menu or simulating real-world interactions, you can perform a right-click using the button: ‘right’ option in the click() method.
Related Articles
- How to Select DropDown Value in Playwright Using selectOption()
- How to Type Text in Playwright Using Fill Method
- How to Get the Current Page URL Using page.url() in Playwright
Hi, I’m Aravind, a seasoned Automation Test Engineer with 17+ years of industry experience. I specialize in tools like Selenium, JMeter, Appium, and Excel automation. Through this blog, I share practical tutorials, tips, and real-world insights to help testers and developers sharpen their automation skills.