Last updated on August 1st, 2025 at 04:31 am
In this quick tutorial, I’ll show you how the
fill()
method works, where to use it, and a few tips to avoid common mistakes.
- What is the fill() Method in Playwright?
- Basic Example: Typing Text into an Input Field
- Basic Playwright Tutorial Quick Links
- Real-Life Example: Filling a Registration Form
- Common errors to avoid while using the fill() method
- fill() vs type(): What's the Difference?
- Fill and press Enter in Playwright
- Fill Hidden Input in Playwright
- Final Thought
If you are starting with playwright automation testing, you’ll first want to learn how to type text into input fields. You can use Playwright’s fill() method to fill the text fields of a login form, a search bar, or a registration page.
What is the fill() Method in Playwright?
One can use the fill() method to enter text into an input field or any element that supports text entry. The fill() method will first clear any existing value and then type the new text you provide.
Syntax of fill() method
await page.fill(selector, text);
- selector: A selector that identifies the input field.
- text: The text you want to type.
Basic Example: Typing Text into an Input Field
Consider you have the following HTML input field:
<input type="text" id="searchField" placeholder="Enter search term">
Here’s how you can type “Playwright Automation” into that input using Playwright:
await page.fill('#searchField', 'Playwright Automation');
That’s it! The playwright will:
- Locate the element using #searchField
- Clear any existing text (if any)
- Type “Playwright Automation” into the input textbox using the fill() method.
Basic Playwright Tutorial Quick Links
- Get Page Title Using page.title()
- Click a Button Using the click() Method
- Simulate the Right Click Using the click() method
- Select Checkboxes Using check() and setChecked() Methods
- Clear Input Text Field Value in Playwright
Real-Life Example: Filling a Registration Form
Here’s a complete example where we use the fill() method to fill the registration form’s input text fields:
const { test, expect } = require('@playwright/test');
test('Example to input text using fill() method in Playwright', async ({ page }) => {
await page.goto('https://only-testing-blog.blogspot.com/2014/05/form.html');
await page.locator('input[name="FirstName"]').fill('Liam');
await page.locator('input[name="LastName"]').fill('Smith');
await page.locator('input[name="EmailID"]').fill('youremail@youremail.com');
await page.locator('input[name="MobNo"]').fill('1111111111');
await page.locator('input[name="Company"]').fill('Your company name');
await page.getByRole('button', { name: 'Submit' }).click();
});
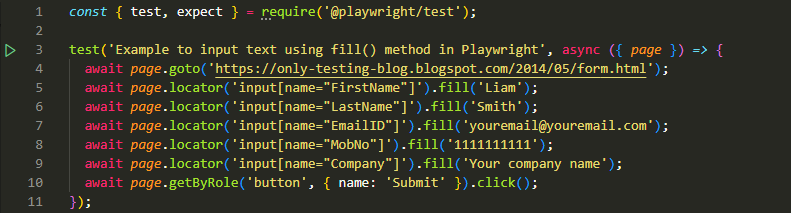
Common errors to avoid while using the fill() method
If the playwright’s fill() method is not working, then there can be any of the reasons given below.
- Element not visible: Make sure that the input field is visible on the page before you type text using the fill() method, or you’ll get an error.
- Page not loaded yet: Always wait for the page or element to load before using fill().
- You can use await page.waitForSelector(‘#username’); before filling the input.
- Use fill() only for inputs: If you’re trying to simulate typing character-by-character, use the .type() method instead of fill().
fill() vs type(): What’s the Difference?
Here is a difference between the fill() method and the type() method in Playwright.
Method | Behavior | Use Case |
fill() | Clears existing text, then types | Best for replacing whole text |
type() | Types character by character | Type character by character |
In short, you can use the fill() method to type whole text and the type() method to type character by character.
Syntax of the type() method in Playwright
await page.type('#APjFqb', 'Playwright tutorial');
Fill and press Enter in Playwright
You can use the page.fill() and page.press() methods to simulate filling and pressing the Enter key action.
Example of Fill and Press Enter
Let’s say you have this simple HTML:
<input type="text" id="searchBox" placeholder="Search...">
You can use the code given below to fill it with a search term and press Enter using Playwright:
await page.fill('#searchBox', 'Playwright tutorial');
await page.press('#searchBox', 'Enter');
Code Breakdown:
- page.fill() will type the text into the input search box.
- page.press() simulates a keyboard press (in this case, the Enter key).
Fill Hidden Input in Playwright
Sometimes, you may need to interact with hidden input elements, such as hidden inputs used in forms for tracking or IDs, or to upload a custom file.
By default, page.fill() does not work on hidden elements. If an input field is hidden (e.g., display: none, visibility: hidden, or type=”hidden”), then Playwright will throw an “Element is not visible” error.
You can use the evaluate() method to set the value directly in a hidden input field.
Let’s say you have below given HTML code for the hidden input field.
<input type="hidden" id="userId" value="123">
Here is how you can fill a hidden field using the evaluate() method in Playwright.
await page.evaluate(() => {
document.querySelector('#userId').value = '456';
});
Final Thought
You can use the fill() method in playwright to fill the input text or text area. If you want to type character by character, then you can use the type() method. You can simulate keypress actions using the press() method. The evaluate() function will help you to fill in hidden input fields.
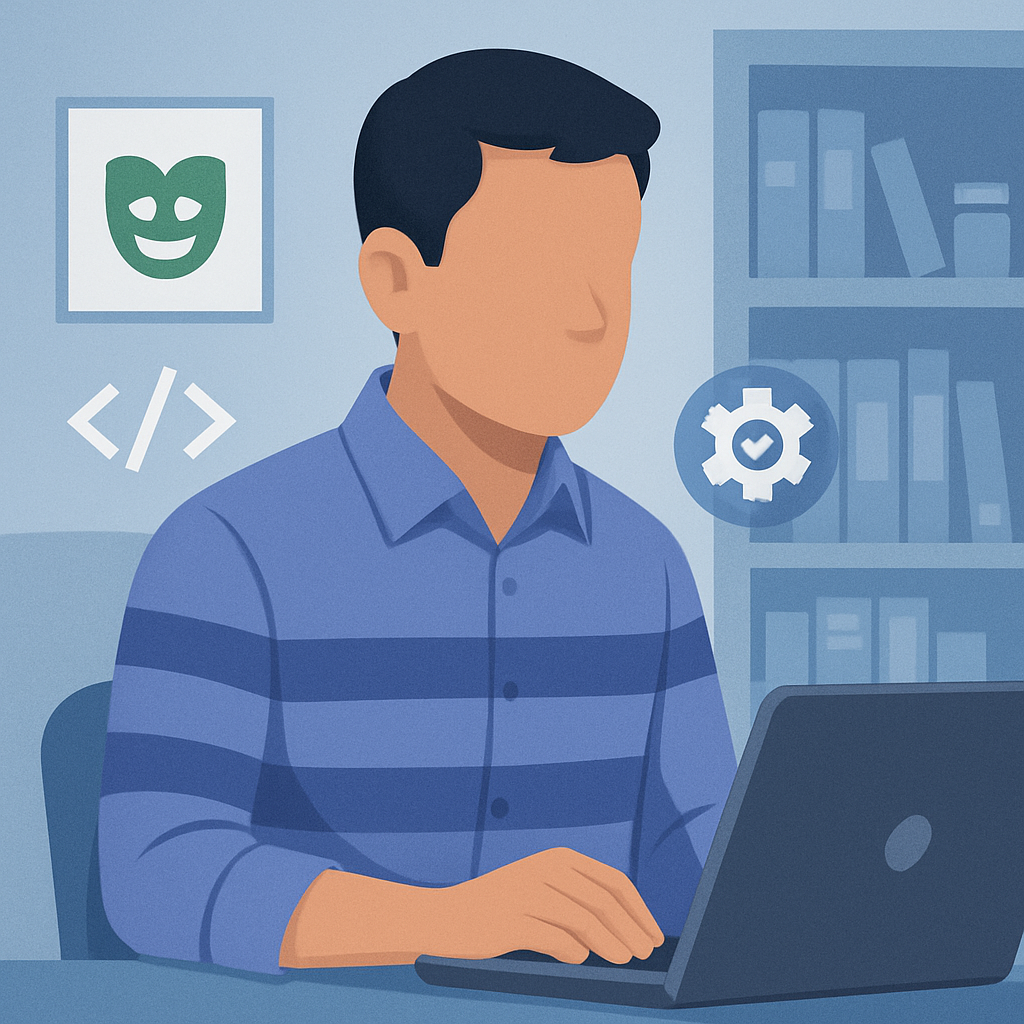
Hi, I’m Aravind — a seasoned Automation Test Engineer with over 17 years of hands-on experience in the software testing industry. I specialize in tech troubleshooting and tools like Selenium, Playwright, Appium, JMeter, and Excel automation. Through this blog, I share practical tutorials, expert tips, and real-world insights to help testers and developers improve their automation skills.In addition to software testing, I also explore tech trends and user-focused topics, including Snapchat guides, codeless test automation, and more.