Last updated on June 25th, 2025 at 04:05 am
This guide will show you how to scroll in Playwright, including how to scroll down, up, or to a specific element. You’ll learn different scrolling techniques using built-in methods and JavaScript execution for smooth and reliable automation.
Playwright provides elegant methods for performing scrolling actions in automation scripts. To scroll to a specific element, you can use the scrollIntoViewIfNeeded()
method. If you need to scroll by a specific number of pixels, the mouse.wheel(x, y)
method is useful. Additionally, you can use the window.scrollTo(x, y)
method to scroll to a particular position on the page, such as the top or bottom.
This Playwright scrolling guide will walk you through all the scrolling techniques available in Playwright.
Why Scrolling Matters in Test Automation
Web applications developed in modern technologies frequently use:
- Lazy-loaded content
- Infinite scroll pages
- Dynamic elements that appear on scroll
- Fixed headers that require scrolling to access content
In Playwright automation, interacting with elements that load upon scrolling requires correctly handling the scroll behavior.
Basic Scrolling Methods in Playwright
There are several ways to perform scrolling in Playwright, depending on your test automation needs. Let’s go through each scrolling method one by one with examples.
Scrolling to an Element Using scrollIntoViewIfNeeded()
If you want to scroll to an element on a web page, you can use the scrollIntoViewIfNeeded() method. This method scrolls the page up or down to bring the element into view, depending on its position.
Here’s a clean and complete way to present that with an example:
Example: Scroll to an Element in Playwright using scrollIntoViewIfNeeded()
import { test, expect } from '@playwright/test';
test('Scroll in to view Playwright example', async ({ page }) => {
await page.goto('https://only-testing-blog.blogspot.com/2025/04/alert-dialogs.html');
// Scrolls to the element if it's not in view
const element = await page.locator('.flatpickr-input');
await element.scrollIntoViewIfNeeded();
await page.waitForTimeout(2000);
// You can now interact with the element
await element.click();
});
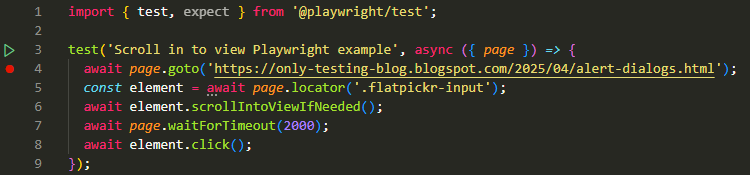
Code Breakdown
- The scrollIntoViewIfNeeded() method will scroll to the element if it is not available in view.
- After scrolling, it will click on an element using the click() method.
Scroll by Pixel Using mouse.wheel(x,y) Method
Playwright allows scrolling both horizontally and vertically by pixels using the mouse.wheel(x, y) method. You can specify the x and y pixel values as needed to control the scroll direction and distance.
Here is an example to scroll down and up by pixels in Playwright
Example: Scroll down/up by Pixels Using the mouse.wheel(x,y) method
import { test, expect } from '@playwright/test';
test('Scroll by pixel example in Playwright', async ({ page }) => {
await page.goto('https://only-testing-blog.blogspot.com/2025/04/alert-dialogs.html');
//Scroll down by 500 pixel.
await page.mouse.wheel(0, 500);
await page.waitForTimeout(2000);
//Scroll up by 500 pixel.
await page.mouse.wheel(0, -500);
await page.waitForTimeout(2000);
});
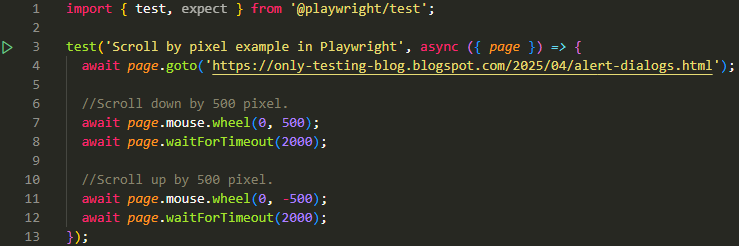
Code Breakdown
- mouse.wheel(0, 500) method will scroll down by 500 pixels.
- mouse.wheel(0, -500) method will scroll up by 500 pixels.
Scroll to Page Coordinates Using the scrollTo(x,y) Method
If you want to scroll to specific coordinates on a page, you can use the scrollTo(x, y) method. Provide the x and y values to scroll horizontally or vertically as needed.
Example: Scroll to coordinates using the scrollTo(x,y) method
import { test, expect } from '@playwright/test';
test('Scrolling to Page Coordinates', async ({ page }) => {
await page.goto('https://only-testing-blog.blogspot.com/2025/04/alert-dialogs.html');
//Scroll to page coordinates
await page.evaluate(() => window.scrollTo(0, 1000));
await page.waitForTimeout(2000);
});
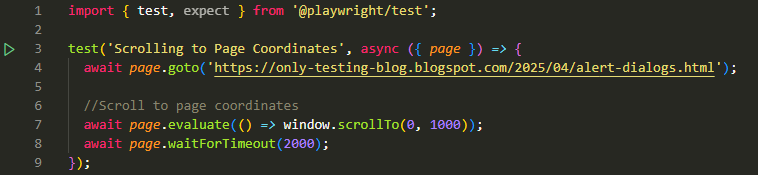
Code Breakdown
- The window.scrollTo(0, 1000) method will scroll down the page by 1000 pixels.
Scroll to the Bottom and Top of the Page in Playwright
Scrolling to the top or bottom of a page is simple in Playwright. You can use the scrollTo(x, y) method to scroll to the desired position—use y = 0 to scroll to the top, or a large y value (like document.body.scrollHeight) to scroll to the bottom.
Example: Scroll to the top/bottom of the page
import { test, expect } from '@playwright/test';
test('Scroll to top and bottom of the page in Playwright Example', async ({ page }) => {
await page.goto('https://only-testing-blog.blogspot.com/2025/04/alert-dialogs.html');
//Scroll to bottom of the page.
await page.evaluate(() => {
window.scrollTo(0, document.body.scrollHeight);
});
await page.waitForTimeout(2000);
//Scroll to top of the page.
await page.evaluate(() => {
window.scrollTo(0, 0);
});
await page.waitForTimeout(2000);
});
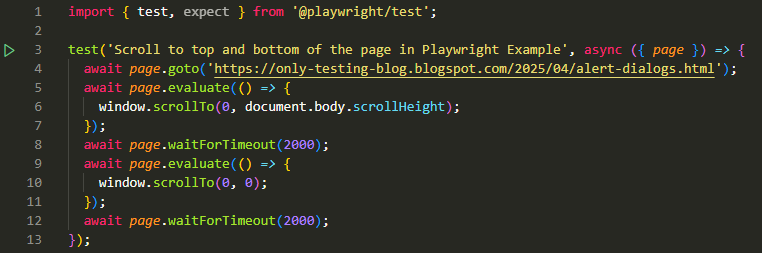
Code Breakdown
- scrollTo(0, document.body.scrollHeight) will scroll to the bottom of the page.
- window.scrollTo(0, 0) will scroll to the top of the page.
Final Thoughts
You can create a robust Playwright automation test case by accurately simulating user interactions with modern web applications. Use Playwright’s comprehensive scrolling API to handle scrolling interactions in your test automation. With techniques and functions outlined in this guide, you’ll ensure your Playwright tests reliably interact with all parts of your web application, regardless of their position on the page.
Related Articles
- How to Handle Date Pickers in Playwright with Examples
- How to Handle Dialog Box in Playwright With Example
- How to Select Checkboxes in Playwright: A Complete Guide
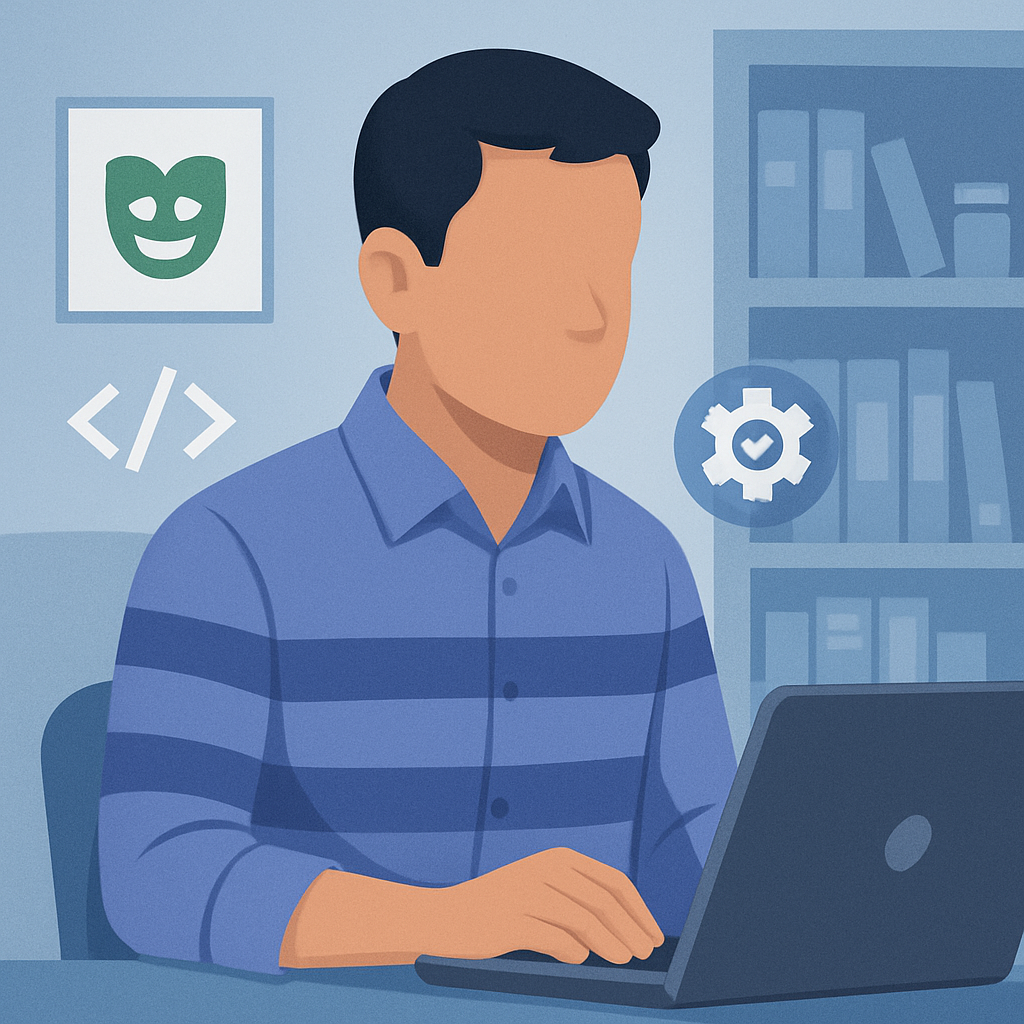
Hi, I’m Aravind — a seasoned Automation Test Engineer with over 17 years of hands-on experience in the software testing industry. I specialize in tech troubleshooting and tools like Selenium, Playwright, Appium, JMeter, and Excel automation. Through this blog, I share practical tutorials, expert tips, and real-world insights to help testers and developers improve their automation skills.In addition to software testing, I also explore tech trends and user-focused topics, including Snapchat guides, codeless test automation, and more.