Dialog boxes, such as alerts, confirmations, and prompts, are common elements of web applications. Handling Dialog boxes smoothly in Playwright automation is one crucial task. Playwright provides simple yet powerful methods for interacting with dialog boxes.
This guide teaches you about the different types of dialog boxes in Playwright, how to handle alerts, confirms, and prompts, and provides practical examples with code snippets.
You can use dialog.accept() method to accept(click OK) and dialog.dismiss() method to dismiss(click cancel) the alert, confirm and prompt dialog.
Types of Dialog Boxes in Playwright
A playwright can handle the following dialog types:
- Alert – A simple pop-up with a message and an OK button.
- Confirm – A dialog with OK and Cancel buttons.
- Prompt – A dialog that accepts user input.
Handling Dialog Boxes in Playwright
You can use the page.on(‘dialog’) event listener to handle different dialog boxes in Playwright.
Let’s see how to handle a simple alert, confirmation dialog, and prompt dialog in Playwright.
Handling Simple Alert Dialog in Playwright
An alert dialog contains an OK button only. You can use dialog.accept() method to accept the alert.
Example of handling an Alert in Playwright
const { test, expect } = require('@playwright/test');
test('Handle simple alert dialog in playwright', async ({ page }) => {
await page.goto('https://only-testing-blog.blogspot.com/2025/04/alert-dialogs.html');
page.on("dialog", async (dialog) => {
//Verify alert type.
expect(dialog.type()).toContain("alert");
//Verify alert message text.
expect(dialog.message()).toContain("This is an Alert box!");
//Accept alert.
await dialog.accept();
});
await page.getByRole('button', { name: 'Alert' }).click();
await page.waitForTimeout(3000);
});
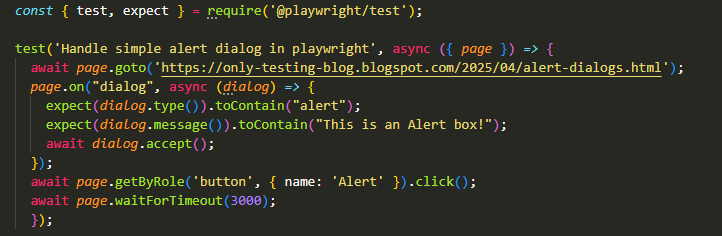
Code Breakdown
- First of all, we open the test page URL.
- Used page.on(“dialog”) event listener to listen to the event.
- Clicked on the Alert button to generate an alert.
- Verified dialog type using expect(dialog.type()).toContain()
- Verified alert text using expect(dialog.message()).toContain()
- Accepted alert using await dialog.accept()
Handling Confirm Dialog in Playwright
A confirmation dialog has OK and Cancel buttons. You can click on OK to accept and Cancel to dismiss it.
Let’s learn how to accept and dismiss confirmation in the Playwright test.
Example of accepting confirmation in Playwright
const { test, expect } = require('@playwright/test');
test('Accept(click OK) confirmation dialog', async ({ page }) => {
await page.goto('https://only-testing-blog.blogspot.com/2025/04/alert-dialogs.html');
page.on("dialog", async (dialog) => {
//Verify alert dialog type.
expect(dialog.type()).toContain("confirm");
//Verify confirm dialog message text.
expect(dialog.message()).toContain("Do you want to proceed?");
//Accept confirm dialog.
await dialog.accept();
});
await page.getByRole('button', { name: 'Confirm' }).click();
await page.waitForTimeout(3000);
});
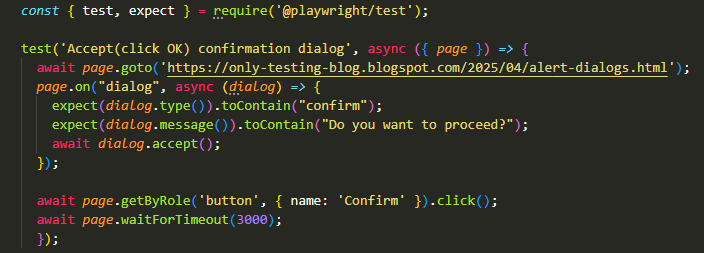
Code Breakdown
- Verified dialog type using expect(dialog.type()).toContain()
- Verified confirm dialog message text using expect(dialog.message()).toContain()
- Accepted confirmation using
await dialog.accept()
Example of dismissing confirmation in Playwright
If you want to dismiss the confirmation, you can use the dialog.dismiss() method as given in the example below.
const { test, expect } = require('@playwright/test');
test('Dismiss(click cancel) confirmation dialog', async ({ page }) => {
await page.goto('https://only-testing-blog.blogspot.com/2025/04/alert-dialogs.html');
page.on("dialog", async (dialog) => {
expect(dialog.type()).toContain("confirm");
expect(dialog.message()).toContain("Do you want to proceed?");
//Dismiss confirm dialog text.
await dialog.dismiss();
});
await page.getByRole('button', { name: 'Confirm' }).click();
await page.waitForTimeout(3000);
});
Code Breakdown
- Dismissed (click cancel) confirm dialog using await dialog.dismiss()
Handling Prompt Dialog in Playwright
The prompt dialog contains an input textbox(to send data), OK, and Cancel buttons.
Example of accepting a prompt dialog in Playwright
The following example will verify the dialog type, alert text message, input text string, and accept(click on OK) prompt dialog with input text string.
const { test, expect } = require('@playwright/test');
test('Accept(click OK) Prompt dialog', async ({ page }) => {
await page.goto('https://only-testing-blog.blogspot.com/2025/04/alert-dialogs.html');
page.on("dialog", async (dialog) => {
//Verify prompt dialog type.
expect(dialog.type()).toContain("prompt");
//Verify prompt dialog message text.
expect(dialog.message()).toContain("Please enter your city:");
//Verify default value of input text.
expect(dialog.defaultValue()).toContain("New York");
//Accept confirm dialog with user input text.
await dialog.accept("London");
});
await page.getByRole('button', { name: 'Prompt' }).click();
await page.waitForTimeout(3000);
});
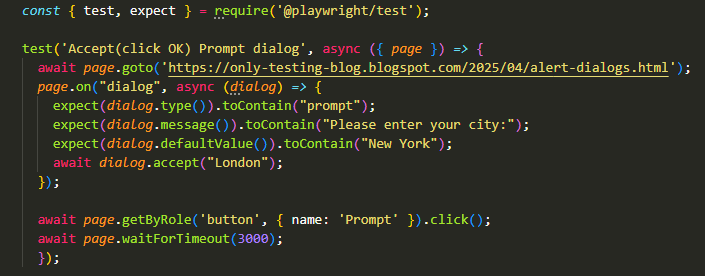
Code Breakdown
- Open the test URL.
- Listen to the prompt alert using the page.on(“dialog”).
- Verify prompt dialog message using expect(dialog.message()).toContain()
- Verify default input text value using expect(dialog.defaultValue()).toContain()
- Type text “London” in the input text field and accept the alert using await dialog.accept()
Best Practices for Handling Dialogs in Playwright
- Always handle dialogs – Unhandled dialogs can block test execution.
- Use assertions – Verify dialog messages for correctness.
- Mock dialogs in tests – Override default behavior for predictable tests.
Final Thoughts
Handling dialog boxes in Playwright is straightforward with the page.on(‘dialog’) event listener. Whether it’s an alert, confirm, or prompt, you can use methods like accept() and dismiss() in Playwright to interact with them seamlessly.
Related Articles
- How to Select Checkboxes in Playwright: A Complete Guide
- How to Handle Tables in Playwright: A Comprehensive Guide
- How to Perform a Double Click in Playwright Using dblclick()
Hi, I’m Aravind, a seasoned Automation Test Engineer with 17+ years of industry experience. I specialize in tools like Selenium, JMeter, Appium, and Excel automation. Through this blog, I share practical tutorials, tips, and real-world insights to help testers and developers sharpen their automation skills.