In this article, we will learn how to use toHaveTitle() assertion method in Playwright to verify and assert title of the page with example.
Verifying the page title before performing any action in Playwright end-to-end automation testing is important. Using page title verification, you can make sure that the correct page is loaded to perform further testing actions. Playwright provides a built-in toHaveTitle() assertion method to verify and assert the title.
What is toHaveTitle() in Playwright?
In Playwright, toHaveTitle() is a powerful assertion method provided by the Playwright Test Runner. You can use the toHaveTitle() assertion to verify whether the actual page title matches the expected title.
Playwright toHaveTitle() Syntax
Here is a syntax to use toHaveTitle() in a Playwright automation test.
Syntax to assert page title:
await expect(page).toHaveTitle('Expected Page Title');
The above syntax will check and compare the current loaded page’s title with the expected text string.
You can use a regular expression as well to match the page title.
Syntax to assert page title using RegExp:
await expect(page).toHaveTitle(/Example/);
The above syntax will assert the page title using a regular expression.
You can use page.title() to get the title of the page in Playwright.
Example: Using toHaveTitle() in Playwright Test
Here is a complete example to check the title of a webpage using toHaveTitle.
JavaScript / TypeScript Example
const { test, expect } = require('@playwright/test');
test('Verify page title using toHaveTitle', async ({ page }) => {
await page.goto('https://example.com');
await expect(page).toHaveTitle("Example Domain") // Assert the exact title
});
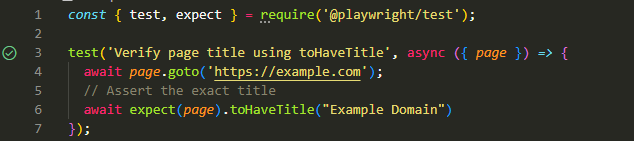
Code Breakdown
- import { test, expect } from ‘@playwright/test’: It will import Playwright’s test and assertion functions.
- await page.goto(…): Open website URL.
- await expect(page).toHaveTitle(…): Assert the page title using toHaveTitle
Example with Regular Expression
If a page title is dynamically changing, you can use a regular expression to match part of the title.
const { test, expect } = require('@playwright/test');
test('Verify page title using toHaveTitle', async ({ page }) => {
await page.goto('https://example.com');
//Assert title using RegExp
await expect(page).toHaveTitle(/Example/);
});
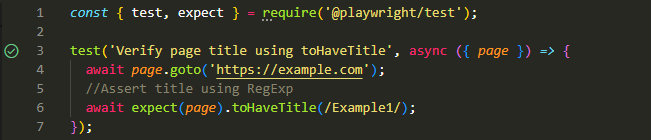
The above example will match any title containing “Example”, like Example Domain, My Example Site, Welcome to Example, etc.
What If the Title Doesn’t Match?
If the title does not match, toHaveTitle() will fail. The playwright will retry for a few seconds (auto-wait) and then throw an error like:
Error: Timed out 5000ms waiting for expect(locator).toHaveTitle(expected)
Locator: locator(':root')
Expected string: "Example Domain"
Received string: "Some other page title"
You can see this error log in the terminal as well as in the HTML report(npx playwright show-report).
Important Notes While Using toHaveTitle()
- toHaveTitle() waits automatically for the title to appear — no need to manually add waitForLoadState()
- This assertion is only available with Playwright’s test runner, not with standalone scripts using plain Playwright
Final Thoughts
Using toHaveTitle() in Playwright is the easiest way to ensure you’re on the right page. Whether you’re writing functional tests, checking navigation, or validating SEO titles, this built-in assertion is powerful and beginner-friendly.
Don’t forget: combining toHaveTitle with other assertions like toHaveURL() or toHaveText() can help you build more reliable end-to-end tests.
Hi, I’m Aravind, a seasoned Automation Test Engineer with 17+ years of industry experience. I specialize in tools like Selenium, JMeter, Appium, and Excel automation. Through this blog, I share practical tutorials, tips, and real-world insights to help testers and developers sharpen their automation skills.