In this post, I’ll break down what await really does, how it works with async, and when to use Promise.all in your Playwright test scripts — all explained in simple terms with real examples.
If you’re new to Playwright or are still getting comfortable with async programming in JavaScript, you might be wondering: Why do we write await before nearly every Playwright command?
JavaScript executes code synchronously by default. In Playwright automation test, the `await` keyword is used to pause execution until an asynchronous operation (a Promise) is completed before moving on to the next line of code.
Many Playwright commands, like navigating to a page, clicking a button, or retrieving text, are asynchronous and return a Promise.
If you forget to use `await` before the syntax in Playwright tests, your code may begin executing the next statement before the previous action is complete, which can lead to flaky or broken tests.
What is await in Playwright?
In simple terms:
- await will tell the playwright to pause execution until the execution of the current syntax(like page.click()) is completed.
- await is used with async functions, so your test function must be declared with async.
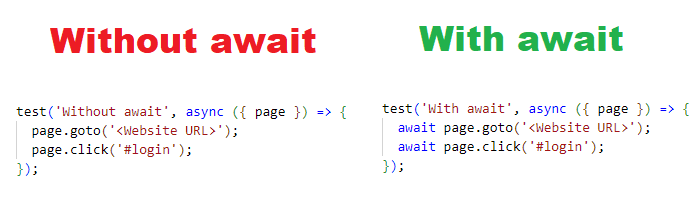
Playwright commands like page.goto(), page.click(), and page.fill() are asynchronous. That means they don’t complete instantly; they return a Promise, which is a placeholder for a future value.
That’s where await comes in.
await tells JavaScript: “Hold on. Don’t move to the next line until this step finishes.”
So, when you write this:
await page.goto('<Website URL>');
You’re saying: “Wait until the page is fully loaded before doing anything else.”
Why You Should Use await in Playwright
Here’s what happens if you don’t use await:
page.goto('<Website URL>');
page.click('#login');
This might fail. Why? Because page.click() might run before the page is loaded — and the element isn’t there yet.
Now compare it with this:
await page.goto('<Website URL>');
await page.click('#login');
Now you’re waiting for each step to complete. Much more reliable and less flaky.
When to Use await in Playwright
Here’s a quick list of when you should use await in Playwright:
Use await With… | Reason |
page.goto() | Wait for the page to fully load |
page.click() | Wait for the click to finish |
page.fill() | Wait for the input to be filled |
page.waitForSelector() | Wait for an element to appear |
page.locator().click() | Waits for the action on locator |
Any Promise-returning method | Waits for the action on the locator |
If the method is asynchronous (returns a Promise), use await.
When Not to Use await in Playwright
There are some cases where await is not required:
Don’t Use await With… | Why |
Defining a locator: page.locator(‘#id’) | This is synchronous — it doesn’t interact with the page |
Plain variable assignments: const x = 5 | Not async |
Inside Promise.all([]) | await is used once outside the array, not before each function |
Example of no await needed:
const username = page.locator('#username'); // no await here
await username.fill('testuser'); // await here
Playwright Async + Await = Reliable Tests
To use await, your test function must be marked as async.
That’s why almost every Playwright test looks like this:
test('Login', async ({ page }) => {
await page.goto('<Website URL>');
await page.fill('#username', 'testuser');
await page.fill('#password', 'testpassword');
await page.click('#submit');
});
- async allows you to use await inside the function.
- await ensures steps run one after the other, not all at once.
Use Promise.all() for Parallel Actions in Playwright
Want to run two things at once — like clicking and waiting for navigation? Use Promise.all().
Here’s how you can do that:
await Promise.all([
page.waitForNavigation(),
page.click('#next-page')
]);
This tells Playwright:“Start both actions, and move on only when both are done.”
When to use Promise.all in Playwright:
- Clicking a button and waiting for navigation.
- Submitting a form and waiting for the page to update.
- Triggering multiple async tasks you want to run together.
Summary: await, async, and Promise.all() in Playwright
Concept | What It Does |
await | Waits for a Promise to finish before moving on |
async | Declares a function that can use await |
Promise.all() | Waits for multiple async actions to complete together |
Final Thoughts
Use await for every Playwright action that touches the page, like clicking, filling, navigating, or waiting for elements. Skip await for things like defining locators, constants, or when you’re not calling a Promise-returning method. Get into the habit of using await wisely and your tests will become more stable, predictable, and easier to debug.
Hi, I’m Aravind, a seasoned Automation Test Engineer with 17+ years of industry experience. I specialize in tools like Selenium, JMeter, Appium, and Excel automation. Through this blog, I share practical tutorials, tips, and real-world insights to help testers and developers sharpen their automation skills.